437. Path Sum III
1. Question
Given the root
of a binary tree and an integer targetSum
, return the number of paths where the sum of the values along the path equals targetSum
.
The path does not need to start or end at the root or a leaf, but it must go downwards (i.e., traveling only from parent nodes to child nodes).
2. Examples
Example 1:
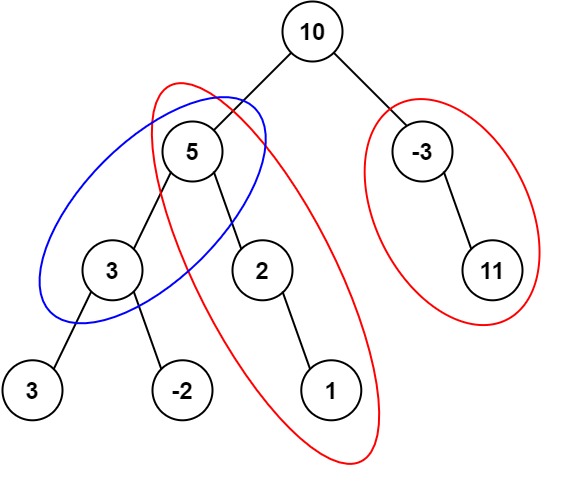
Input: root = [10,5,-3,3,2,null,11,3,-2,null,1], targetSum = 8
Output: 3
Explanation: The paths that sum to 8 are shown.
Example 2:
Input: root = [5,4,8,11,null,13,4,7,2,null,null,5,1], targetSum = 22
Output: 3
3. Constraints
- The number of nodes in the tree is in the range
[0, 1000]
. - -109 <= Node.val <= 109
-1000 <= targetSum <= 1000
4. References
来源:力扣(LeetCode) 链接:https://leetcode-cn.com/problems/path-sum-iii 著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
5. Solutions
这道题需要两个递归,第一个递归是以某个节点开始每个都当作单独的树。第二个是在第一个的基础上进行dfs。
class Solution {
public int pathSum(TreeNode root, int targetSum) {
if (root == null) {
return 0;
}
return getCount(root, targetSum) + pathSum(root.left, targetSum) + pathSum(root.right, targetSum);
}
private int getCount(TreeNode root, int sum) {
int res = 0;
if (root == null) {
return res;
}
if (root.val == sum) {
res++;
}
return res += getCount(root.left, sum - root.val) + getCount(root.right, sum - root.val);
}
}